Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 선언형UI
- ImageView
- 클린 아키텍처
- Android 애드몹
- 컴포넌트
- thread
- 안드로이드 라이브러리
- 안드로이드광고
- android kakao map
- android daum map
- HTTP
- RecyclerView
- android 지도
- Firebase
- 안드로이드 카카오 지도
- 아키텍처
- Android
- dynamiclink
- 애드몹광고
- 다이나믹 링크
- 안드로이드컴포즈
- 젯팩컴포즈
- 안드로이드
- JetpackCompose
- 파이어베이스
- component
- 애드몹배너
- glide
- 동적 링크
- Clean Architecture
Archives
- Today
- Total
코딩스토리
[Android/안드로이드] RecyclerView ItemDecoration으로 아이템 항목 구분지어주기 본문
앱을 구현할 때 디자인에 따라 마지막 아이템만 구분선이 없거나 첫번째 아이템만 구분선이 있는 등 각 항목마다 구분을 지어줄 필요가 있는 디자인이 있습니다.
ItemDecoration을 잘만 사용하게 되면 다양하게 항목을 꾸밀 수 있습니다. ItemDecoration에는 3개의 함수를 제공해주고있습니다.
- onDraw : 항목을 배치하기 전에 호출합니다
- onDrawOver : 모든 항목이 배치된 후에 호출됩니다.
- onItemOffsets : 각 항목을 배치할 때 호출됩니다.
우선 구분선을 만들어준 drawable파일을 만들어보겠습니다.
line_divider.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<size
android:width="1dp"
android:height="1dp" />
<solid android:color="#000000" />
</shape>
MyItemDecoration.java
package com.lakue.recyclerviewmanager;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Rect;
import android.graphics.drawable.Drawable;
import android.view.View;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
public class MyItemDecoration extends RecyclerView.ItemDecoration {
Context context;
Drawable mDivider;
public MyItemDecoration(Context context) {
this.context = context;
mDivider = context.getResources().getDrawable(R.drawable.line_divider);
}
@Override
public void getItemOffsets(@NonNull Rect outRect, @NonNull View view, @NonNull RecyclerView parent, @NonNull RecyclerView.State state) {
super.getItemOffsets(outRect, view, parent, state);
outRect.bottom = 100;
}
@Override
public void onDrawOver(@NonNull Canvas c, @NonNull RecyclerView parent, @NonNull RecyclerView.State state) {
super.onDrawOver(c, parent, state);
int left = parent.getPaddingLeft();
int right = parent.getWidth() - parent.getPaddingRight();
int childCount = parent.getChildCount();
for (int i = 0; i < childCount; i++) {
View child = parent.getChildAt(i);
RecyclerView.LayoutParams params = (RecyclerView.LayoutParams) child.getLayoutParams();
int top = child.getBottom() + params.bottomMargin;
int bottom = top + mDivider.getIntrinsicHeight();
mDivider.setBounds(left, top, right, bottom);
mDivider.draw(c);
}
}
}
이제 RecyclerView에 적용을 하면 각 항목마다 구분선을 넣어집니다.
recyclerView.addItemDecoration(new MyItemDecoration(this));
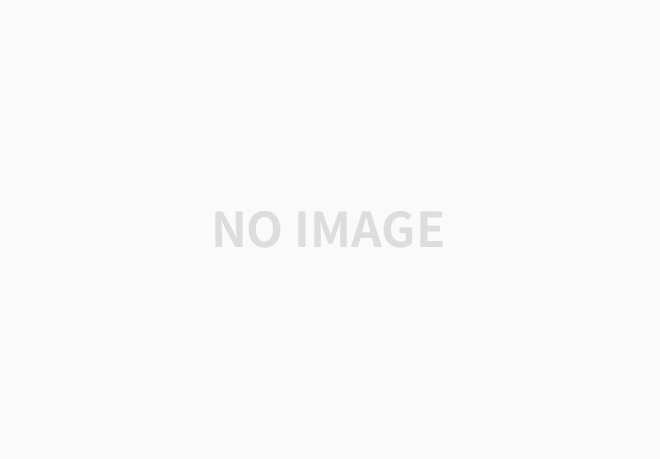
'Android > 유용한 기술' 카테고리의 다른 글
[Android/안드로이드] Kakao 지도 API 연동/카카오 지도 API연동 (4) | 2020.09.15 |
---|---|
[Android/안드로이드] 알람울리기/진동울리기/알림 Notification (0) | 2020.09.13 |
[Android/안드로이드] RecyclerView LayoutManager타입별 사용하기 (0) | 2020.09.11 |
[Android/안드로이드] 키보드로 액티비티 화면 조정 adjustPan (0) | 2020.09.10 |
[Android/안드로이드] 키보드 보이기/키보드 숨기기를 제어하기 (0) | 2020.09.09 |